If instead i is declared as signed, where the overflow semantics are undefined, the compiler has more leeway to use these optimizations. In the next post I will continue our discussion of shared memory by using it to optimize a matrix transpose. For more details refer to the memcpy_async section in the CUDA C++ Programming Guide. For GPUs with compute capability 8.6 maximum shared memory per thread block is 99 KB. An Efficient Matrix Transpose in CUDA C/C++, How to Access Global Memory Efficiently in CUDA C/C++ Kernels, How to Access Global Memory Efficiently in CUDA Fortran Kernels, Top Video Streaming and Conferencing Sessions at NVIDIA GTC 2023, Top Cybersecurity Sessions at NVIDIA GTC 2023, Top Conversational AI Sessions at NVIDIA GTC 2023, Top AI Video Analytics Sessions at NVIDIA GTC 2023, Top Data Science Sessions at NVIDIA GTC 2023. Thedriver will honor the specified preference except when a kernel requires more shared memory per thread block than available in the specified configuration. Weak scaling is often equated with Gustafsons Law, which states that in practice, the problem size scales with the number of processors. // Number of bytes for persisting accesses. Note that the timings are measured on the GPU clock, so the timing resolution is operating-system-independent. Hence, access to local memory is as expensive as access to global memory. For most purposes, the key point is that the larger the parallelizable portion P is, the greater the potential speedup. By understanding how applications can scale it is possible to set expectations and plan an incremental parallelization strategy. Texture memory is also designed for streaming fetches with a constant latency; that is, a cache hit reduces DRAM bandwidth demand, but not fetch latency. For this example, it is assumed that the data transfer and kernel execution times are comparable. For exponentiation with an exponent of 1/3, use the cbrt() or cbrtf() function rather than the generic exponentiation functions pow() or powf(), as the former are significantly faster than the latter. Reproduction of information in this document is permissible only if approved in advance by NVIDIA in writing, reproduced without alteration and in full compliance with all applicable export laws and regulations, and accompanied by all associated conditions, limitations, and notices. .Y stands for the minor version - Introduction of new APIs, deprecation of old APIs, and source compatibility might be broken but binary compatibility is maintained. NVIDIA Ampere GPU Architecture Tuning, 1.4.1.2. In CUDA there is no defined global synchronization mechanism except the kernel launch. This variant simply uses the transpose of A in place of B, so C = AAT. Bfloat16 provides 8-bit exponent i.e., same range as FP32, 7-bit mantissa and 1 sign-bit. Because the default stream, stream 0, exhibits serializing behavior for work on the device (an operation in the default stream can begin only after all preceding calls in any stream have completed; and no subsequent operation in any stream can begin until it finishes), these functions can be used reliably for timing in the default stream. The compiler can optimize groups of 4 load and store instructions. Randomly accessing. Its important to note that both numbers are useful. An application can also use the Occupancy API from the CUDA Runtime, e.g. Data Transfer Between Host and Device provides further details, including the measurements of bandwidth between the host and the device versus within the device proper. Where to Install Redistributed CUDA Libraries, 17.4. To prevent the compiler from allocating too many registers, use the -maxrregcount=N compiler command-line option (see nvcc) or the launch bounds kernel definition qualifier (see Execution Configuration of the CUDA C++ Programming Guide) to control the maximum number of registers to allocated per thread. Both correctable single-bit and detectable double-bit errors are reported. CUDA-GDB is a port of the GNU Debugger that runs on Linux and Mac; see: https://developer.nvidia.com/cuda-gdb. Instead, all instructions are scheduled, but a per-thread condition code or predicate controls which threads execute the instructions. However, for each iteration i, all threads in a warp read the same value from global memory for matrix A, as the index row*TILE_DIM+i is constant within a warp. Certain functionality might not be available so you should query where applicable. Page-locked mapped host memory is allocated using cudaHostAlloc(), and the pointer to the mapped device address space is obtained via the function cudaHostGetDevicePointer(). Because the minimum memory transaction size is larger than most word sizes, the actual memory throughput required for a kernel can include the transfer of data not used by the kernel. Register dependencies arise when an instruction uses a result stored in a register written by an instruction before it. Furthermore, the need for context switching can reduce utilization when work from several contexts could otherwise execute concurrently (see also Concurrent Kernel Execution). This utility allows administrators to query GPU device state and, with the appropriate privileges, permits administrators to modify GPU device state. Starting with CUDA 11.0, devices of compute capability 8.0 and above have the capability to influence persistence of data in the L2 cache. Block-column matrix (A) multiplied by block-row matrix (B) with resulting product matrix (C).. In this case, multiple broadcasts from different banks are coalesced into a single multicast from the requested shared memory locations to the threads. If sequential threads in a warp access memory that is sequential but not aligned with a 32-byte segment, five 32-byte segments will be requested, as shown in Figure 4. The context encapsulates kernel launches and memory allocations for that GPU as well as supporting constructs such as the page tables. Please refer to the EULA for details. You want to sort all the queues before you collect them. For example, the ability to overlap kernel execution with asynchronous data transfers between the host and the device is available on most but not all GPUs irrespective of the compute capability. All rights reserved. Fetching ECC bits for each memory transaction also reduced the effective bandwidth by approximately 20% compared to the same GPU with ECC disabled, though the exact impact of ECC on bandwidth can be higher and depends on the memory access pattern. by synchronization between blocks, i take it that you mean preserve the order of blocks there is at least 1 method that i can think of, that generally accomplishes this you can either push a sequence of block numbers into (global) memory, and have thread blocks base the block they process next on this sequence; the sequence is read via an atomic Device 0 of this system has compute capability 7.0. likewise return their own sets of error codes. A more robust approach is to selectively introduce calls to fast intrinsic functions only if merited by performance gains and where altered behavior can be tolerated. As with the dynamically-linked version of the CUDA Runtime library, these libraries should be bundled with the application executable when distributing that application. The warp wide reduction operations support arithmetic add, min, and max operations on 32-bit signed and unsigned integers and bitwise and, or and xor operations on 32-bit unsigned integers. Sample CUDA configuration data reported by deviceQuery. So, if each thread block uses many registers, the number of thread blocks that can be resident on a multiprocessor is reduced, thereby lowering the occupancy of the multiprocessor. In other words, the term local in the name does not imply faster access. With wrap, x is replaced by frac(x) where frac(x) = x - floor(x). Can anyone please tell me how to do these two operations? Intermediate data structures should be created in device memory, operated on by the device, and destroyed without ever being mapped by the host or copied to host memory. Strong scaling is usually equated with Amdahls Law, which specifies the maximum speedup that can be expected by parallelizing portions of a serial program. Delays in rolling out new NVIDIA drivers could mean that users of such systems may not have access to new features available in CUDA releases. (Consider what would happen to the memory addresses accessed by the second, third, and subsequent thread blocks if the thread block size was not a multiple of warp size, for example.). Devices to be made visible to the application should be included as a comma-separated list in terms of the system-wide list of enumerable devices. Recall that the initial assess step allowed the developer to determine an upper bound for the potential speedup attainable by accelerating given hotspots. vegan) just to try it, does this inconvenience the caterers and staff? A lower occupancy kernel will have more registers available per thread than a higher occupancy kernel, which may result in less register spilling to local memory; in particular, with a high degree of exposed instruction-level parallelism (ILP) it is, in some cases, possible to fully cover latency with a low occupancy. Dynamic parallelism - passing contents of shared memory to spawned blocks? Excessive use can reduce overall system performance because pinned memory is a scarce resource, but how much is too much is difficult to know in advance. Dont expose ABI structures that can change. Some calculations use 10243 instead of 109 for the final calculation. math libraries or deep learning frameworks) do not have a direct dependency on the CUDA runtime, compiler or driver. It enables GPU threads to directly access host memory. For example, if you link against the CUDA 11.1 dynamic runtime, and use functionality from 11.1, as well as a separate shared library that was linked against the CUDA 11.2 dynamic runtime that requires 11.2 functionality, the final link step must include a CUDA 11.2 or newer dynamic runtime. Another, more aggressive, option is -use_fast_math, which coerces every functionName() call to the equivalent __functionName() call. Generally, accessing a register consumes zero extra clock cycles per instruction, but delays may occur due to register read-after-write dependencies and register memory bank conflicts. Often this means the use of directives-based approaches, where the programmer uses a pragma or other similar notation to provide hints to the compiler about where parallelism can be found without needing to modify or adapt the underlying code itself. But this technique is still useful for other access patterns, as Ill show in the next post.). Therefore, in terms of wxw tiles, A is a column matrix, B is a row matrix, and C is their outer product; see Figure 11. It is customers sole responsibility to evaluate and determine the applicability of any information contained in this document, ensure the product is suitable and fit for the application planned by customer, and perform the necessary testing for the application in order to avoid a default of the application or the product. Programmers should be aware of two version numbers. Latency hiding and occupancy depend on the number of active warps per multiprocessor, which is implicitly determined by the execution parameters along with resource (register and shared memory) constraints. NVIDIA-SMI can be used to configure a GPU for exclusive process mode, which limits the number of contexts per GPU to one. \times (4096/8) \times 2 \right) \div 10^{9} = 898\text{GB/s}\). NVIDIA accepts no liability for inclusion and/or use of NVIDIA products in such equipment or applications and therefore such inclusion and/or use is at customers own risk. On discrete GPUs, mapped pinned memory is advantageous only in certain cases. Both the CUDA driver and the CUDA runtime are not source compatible across the different SDK releases. As an exception, scattered writes to HBM2 see some overhead from ECC but much less than the overhead with similar access patterns on ECC-protected GDDR5 memory. For small integer powers (e.g., x2 or x3), explicit multiplication is almost certainly faster than the use of general exponentiation routines such as pow(). These memory spaces include global, local, shared, texture, and registers, as shown in Figure 2. This ensures your code is compatible. We define source compatibility as a set of guarantees provided by the library, where a well-formed application built against a specific version of the library (using the SDK) will continue to build and run without errors when a newer version of the SDK is installed. For example, if the threads of a warp access adjacent 4-byte words (e.g., adjacent float values), four coalesced 32-byte transactions will service that memory access. We can see this usage in the following example: NVRTC is a runtime compilation library for CUDA C++. Computing a row of a tile in C using one row of A and an entire tile of B. Resources stay allocated to each thread until it completes its execution. CUDA kernel and thread hierarchy This is shown in Figure 1. Testing of all parameters of each product is not necessarily performed by NVIDIA. For this reason, ensuring that as much as possible of the data in each cache line fetched is actually used is an important part of performance optimization of memory accesses on these devices. Instructions with a false predicate do not write results, and they also do not evaluate addresses or read operands. If the PTX is also not available, then the kernel launch will fail. OpenCL is a trademark of Apple Inc. used under license to the Khronos Group Inc. NVIDIA and the NVIDIA logo are trademarks or registered trademarks of NVIDIA Corporation in the U.S. and other countries. CUDA reserves 1 KB of shared memory per thread block. These many-way bank conflicts are very expensive. Upgrading dependencies is error-prone and time consuming, and in some corner cases, can even change the semantics of a program. Then, as shown in the figure below, we specify that the accesses to the first freqSize * sizeof(int) bytes of the memory region are persistent. This should be our first candidate function for parallelization. Using a profiler, the developer can identify such hotspots and start to compile a list of candidates for parallelization. If x is the coordinate and N is the number of texels for a one-dimensional texture, then with clamp, x is replaced by 0 if x < 0 and by 1-1/N if 1 Family First Life Compensation,
What Are The Flavors At Kopp's Today,
Verset Biblique Sur La Maman,
Articles C
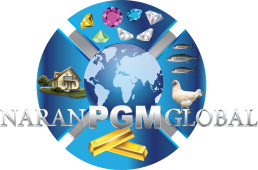